As
we can see, the class in itself it's not very complicated, but this is
also because the panel that it represents is fairly basic. Let's take a
quick look at it:
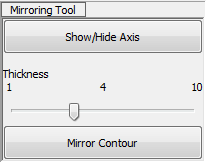
Figure [14]. The graphical distribution of the mirroring panel.
What
we're going to try to achieve in this guide is actually a very similar
panel; it'll have just two buttons, each one of which will have an
associated functionality. We must begin by writing down the class
definition of our new panel, that we'll name interfTestPanel. Following
the pattern shown above, we'll have:
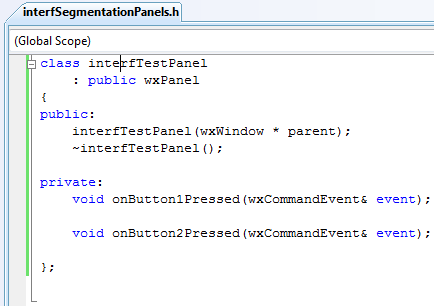
Figure [15]. The declaration of the interfTestPanel class.
As
we can see, we have two methods, onButton1Pressed and onButton2Pressed,
which receive two wxCommandEvent& events. These are the callback
methods that are called once we click on a button.
Now that we have our class definition, we proceed to declare each method of our class.
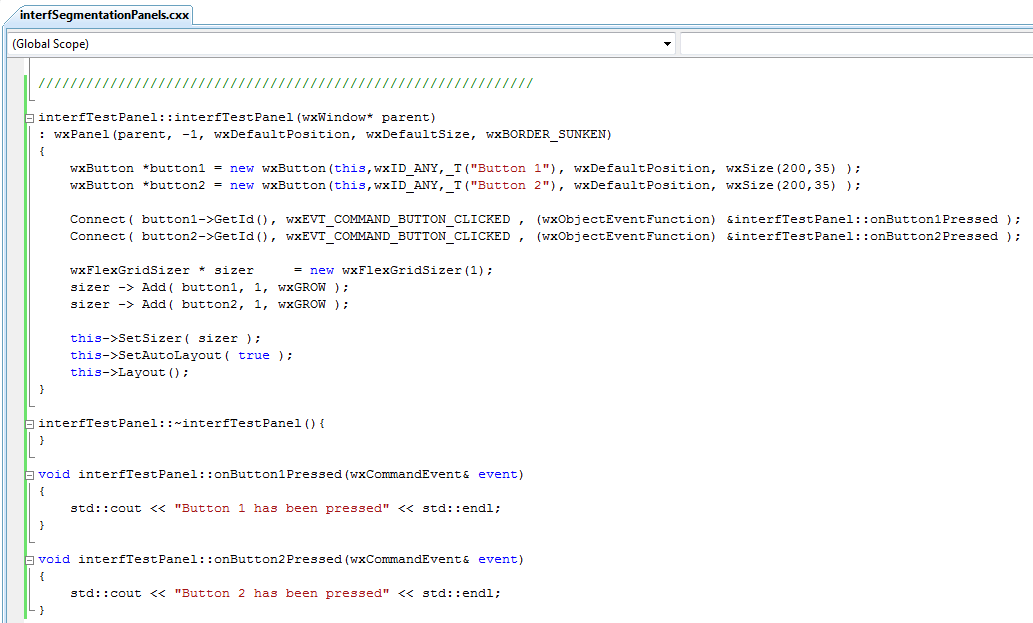
Figure [16]. The declaration of the new interfTestPanel class
The
button callback methods, as we can see, do nothing in particular; they
just print into std::cout a message informing the use that the
respective button's been pressed. Inside the constructor, we create
both buttons as new
wxButtons. These
take as parameters the panel into which they're going to be added, an
ID, a label, and a position. Explaining in detail these parameters (as
well as those used in the call to the
wxPanel constructor) goes beyond the scope of this guide.
We associated an event to each button with the Connect method. In this case it's a
wxEVT_COMMAND_BUTTON_CLICKED event.
Furthermore, each button has an associated callback function, which
must also be specified through the Connect method. The
wxFlexGridSizer which is added immediately afterwards serves as a way to organize the elements on the interface.
Having
done that, we must now make sure that once we click on our button the
panel is added to the interface. In order to make sure of this, we have
to remember the "pipeline" that is followed when we click on a button.
This time around, we will folllow it all the way to the "onTest" method
in
interfMainPanel, as it's
here that we have the necessary tools to display our new panel. Before
getting down to it, we have to add to new parameters to the
interfMainPanel.h file:
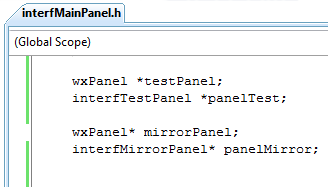
Figure [17]. The declaration of the new panel parameters.
The first parameter is a regular
wxPanel which will contain within it the actual
interfTestPanel, which is the second parameter that's been added. As soon as we have them in our header file, we can go to the
OnTest method and modify it so the panel is shown in the right place. Basing ourselves on the existing
onMirrorPressed method, we get the following code which does exactly what we want it to:
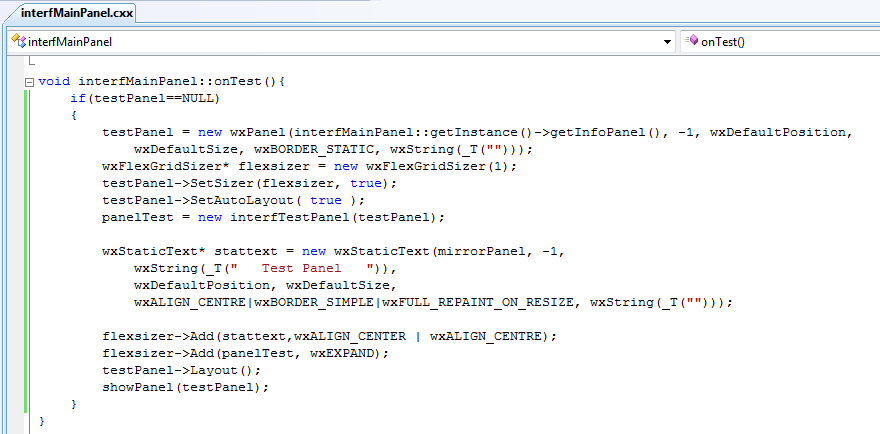
Figure [18]. The fragment of code that serves to show the new panel
What is done here is actually quite simple. The new
testPanel (of object type
wxPanel)
contains within it the panel where the actual test panel is going to be
added. In other words, this is where we will store an instance of the
space underneath the buttons, represented as a panel. Having
created it, we further configure it before creating an instance of the
actual
interfMirrorPanel. This is added to the
wxFlexGridSizer which in turn has been set as the
testPanel'
s Sizer. It is why by doing so we're adding the
interfMirrorPanel to
the space beneath the buttons, which is exactly what we wanted to do!
We end by adding a little bit of text (just for the purpose of staying
close to the other, already existing buttons) and we end by showing our
panel by invoking the
showPanel method and passing it an instance of
testPanel, which now contains within it the
interfMirrorPanel.If we now run the interface and click on the new button, we'll obtain the following result...
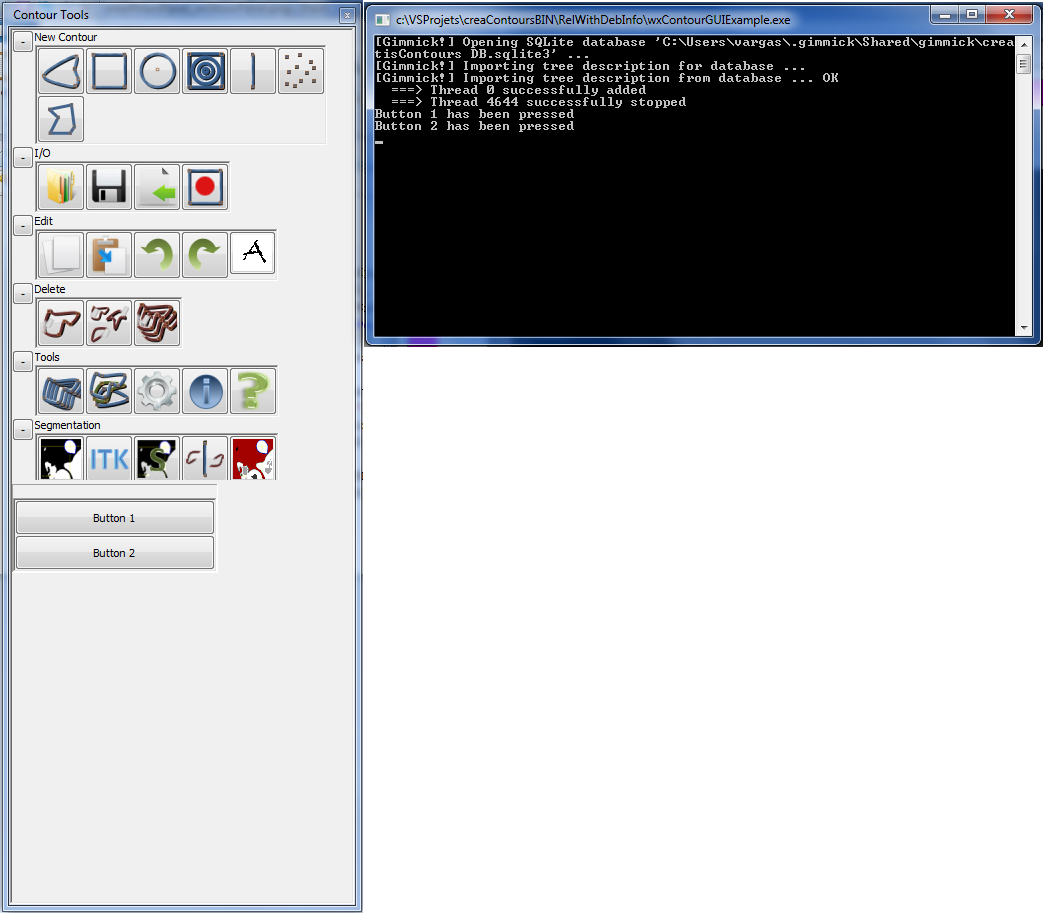
Figure [19]. The resulting panel